So, you’re sitting there, happily coding away, when suddenly you encounter this perplexing error: TypeError: getattr(): attribute name must be string
. It’s like a wild Pokemon appearing out of nowhere. If you’re scratching your head and wondering what went wrong, don’t worry—you’re not alone. This error has puzzled many a coder, but today, we’re going to tame it together.
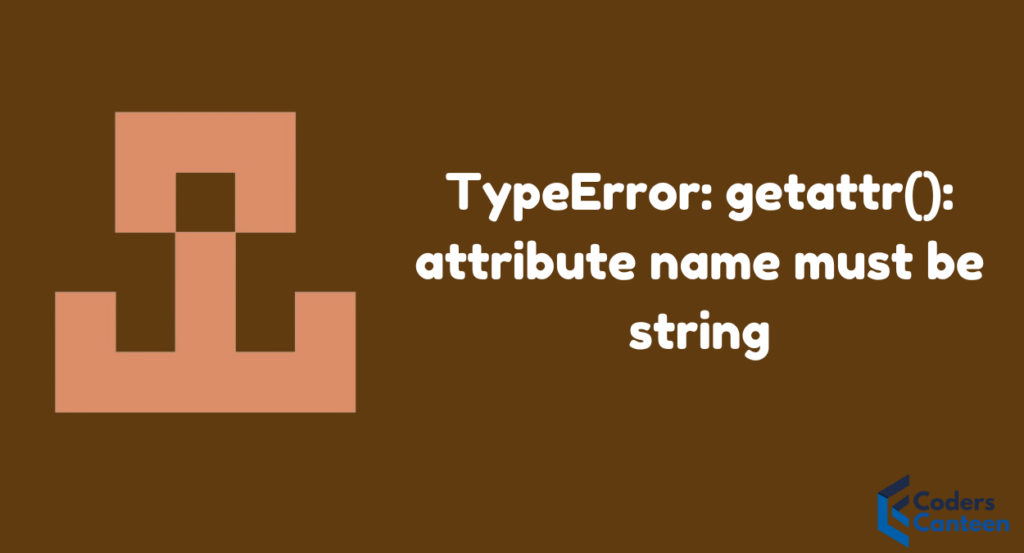
In this article, we’ll break down this error, understand why it happens, and most importantly, how to fix it. We’ll also throw in a bit of humor because, let’s face it, debugging can be frustrating, and a little laughter never hurt anyone. So grab your coffee (or tea, if that’s your jam), and let’s dive in!
Understanding the getattr()
Function
First things first, let’s talk about what the getattr()
function does. In Python, getattr()
is a built-in function that retrieves an attribute from an object. It’s like asking your friend if they have a specific book, and if they do, they hand it over to you. Here’s a basic example:
class Book:
title = "Python Programming"
my_book = Book()
title = getattr(my_book, 'title')
print(title) # Output: Python Programming
In this example, getattr()
is used to get the value of the title
attribute from the my_book
object. Simple, right? But what happens when things go south?
The TypeError
The error message TypeError: getattr(): attribute name must be string
means that somewhere in your code, you’ve passed something other than a string as the attribute name. It’s like asking your friend for a book using sign language when they only understand spoken words. Here’s a scenario that might cause this error:
attr_name = 123 # This should be a string
title = getattr(my_book, attr_name)
In this case, attr_name
is an integer, and getattr()
is not happy about it. Hence, the TypeError
.
Why This Happens
Before we jump into solutions, let’s take a moment to understand why this error occurs. Knowing the “why” can often help prevent similar issues in the future. Here are a few common reasons:
- Typographical Errors: Sometimes, it’s as simple as a typo. You might have intended to pass a string but accidentally passed a different data type.
- Dynamic Attribute Names: If you’re generating attribute names dynamically and something goes wrong in that process, you might end up with a non-string value.
- Misunderstanding Function Behavior: If you’re new to Python or
getattr()
, you might misunderstand how it works and what kind of arguments it expects.
How to Fix It
Now, let’s get to the good stuff—fixing the error. Here are a few strategies you can use.
1. Check Your Attribute Names
The first and most obvious step is to check your attribute names. Make sure they’re strings. This is usually a quick fix.
attr_name = 'title' # Make sure this is a string
title = getattr(my_book, attr_name)
2. Validate Dynamic Attribute Names
If you’re generating attribute names dynamically, add a check to ensure they are strings.
def get_attribute(obj, attr_name):
if not isinstance(attr_name, str):
raise TypeError("Attribute name must be a string")
return getattr(obj, attr_name)
attr_name = 123 # This will raise an error
title = get_attribute(my_book, attr_name)
3. Use Default Values
Sometimes, it’s helpful to provide a default value in case the attribute name is incorrect. This won’t fix the TypeError
, but it can help avoid other potential errors down the line.
title = getattr(my_book, 'title', 'Unknown Title')
print(title) # Output: Python Programming or 'Unknown Title' if attribute is missing
4. Debugging Tips
If you’re still stumped, here are a few additional debugging tips:
- Print Statements: Add print statements to check the type of your attribute name before calling
getattr()
.
print(type(attr_name)) # This should print <class 'str'>
- Try/Except Block: Use a try/except block to catch the error and print a helpful message.
try:
title = getattr(my_book, attr_name)
except TypeError as e:
print(f"An error occurred: {e}")
A Humorous Interlude
Let’s take a break from all the technical talk and inject a bit of humor. Debugging can be like solving a mystery, and sometimes you need to put on your Sherlock Holmes hat. Imagine this conversation with your computer:
You: “Hey, can you get me the title attribute?”
Computer: “Sure, just tell me the attribute name.”
You: “It’s 123.”
Computer: facepalm “I said ‘name’, not ‘number’.”
You: “Oh… right.”
Remember, even the best of us make mistakes, and sometimes you just need to laugh it off and try again.
Real-World Example
Let’s look at a real-world example to solidify our understanding. Suppose you’re building a simple content management system (CMS) where you can dynamically access attributes of content objects.
class Content:
def __init__(self, title, body):
self.title = title
self.body = body
def get_content_attribute(content, attr_name):
if not isinstance(attr_name, str):
raise TypeError("Attribute name must be a string")
return getattr(content, attr_name, 'Attribute not found')
article = Content("Breaking News", "Something happened today...")
try:
print(get_content_attribute(article, 'title')) # Output: Breaking News
print(get_content_attribute(article, 123)) # This will raise a TypeError
except TypeError as e:
print(f"Oops! {e}")
In this example, get_content_attribute()
ensures that the attribute name is a string before attempting to retrieve it. If not, it raises a TypeError
.
Common Mistakes and Misconceptions
Let’s address some common mistakes and misconceptions that can lead to this error:
1. Forgetting the Quotes
One common mistake is forgetting to enclose the attribute name in quotes, especially if you’re hardcoding it.
title = getattr(my_book, title) # Oops! 'title' should be a string
2. Confusing Variable Names and Strings
Sometimes, you might have a variable with the same name as the attribute and forget to pass it as a string.
title = 'title'
print(getattr(my_book, title)) # This works because 'title' is a string
title_var = 'title'
print(getattr(my_book, title_var)) # This also works because title_var is a string variable
3. Misunderstanding the Purpose of getattr()
New Python developers might misuse getattr()
, thinking it does something else entirely. Remember, getattr()
is specifically for retrieving attributes from objects.
Must Read:
- MAFIA Best Understanding in 2024: OpenFlow Statistics (Counters, Timestamps)
- ChatGPT: How to Get the Best Results From ChatGPT in 2024
FAQs
Q: What is getattr()
used for in Python?
A: getattr()
is a built-in Python function used to retrieve the value of an attribute from an object. You provide the object and the attribute name as arguments, and it returns the attribute’s value.
Q: Why do I get a TypeError
with getattr()
?
A: You get a TypeError
with getattr()
when you pass a non-string value as the attribute name. The function expects the attribute name to be a string.
Q: How can I prevent the TypeError
with getattr()
?
A: Ensure that the attribute name you pass to getattr()
is always a string. You can add validation to check the type of the attribute name before calling the function.
Q: Is getattr()
only used for retrieving attributes?
A: Primarily, yes. getattr()
is designed to retrieve attributes from objects. If you need to set or delete attributes, you can use setattr()
and delattr()
respectively.
Conclusion
By now, you should have a solid understanding of the TypeError: getattr(): attribute name must be string
error and how to resolve it. Remember, the key is to ensure that the attribute name you pass to getattr()
is always a string. With the strategies and examples provided in this article, you should be well-equipped to tackle this error and continue your coding journey with confidence.
And next time you encounter an error, just think of it as a quirky puzzle that needs solving. Happy coding!