Python’s Timeit Trouble: In the world of Python programming, when you’re trying to optimize your code’s performance, you often turn to the timeit module. It’s a fantastic tool for measuring the execution time of small code snippets. However, sometimes, when you’re knee-deep in your coding endeavors, you might encounter a rather cryptic error message: “stmt is neither a string nor callable.” So, what exactly does this error mean, and how can you resolve it? Let’s delve into the depths of Python’s timeit module to uncover the answers.
Understanding the Error: Python’s Timeit Trouble
The error message itself might seem perplexing at first glance. It refers to the “stmt” parameter, which is essentially the statement or code snippet you want to time using the timeit module. Now, according to the Python documentation, the “stmt” parameter should either be a string containing the code to be timed or a callable object, such as a function. So, when you encounter the “stmt is neither a string nor callable” error, it means that the parameter you provided doesn’t meet these requirements.
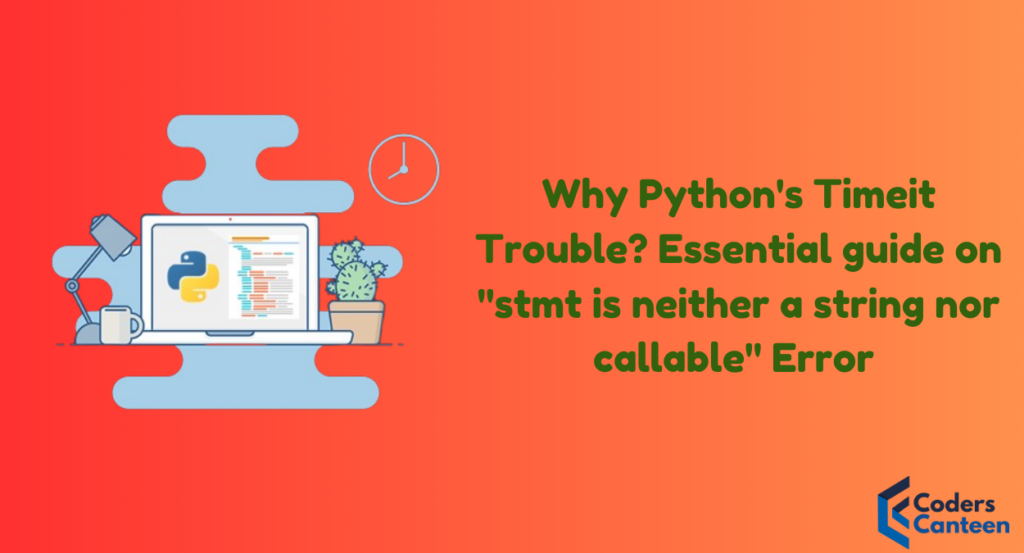
Common Causes of the Error: Python Timeit Trouble
Let’s explore some common scenarios that might lead to this error:
- Missing Quotations: One of the most frequent mistakes is forgetting to enclose your code snippet in quotes when passing it as a string to the timeit function. Without the quotes, Python doesn’t recognize the code as a string, resulting in the error.
- Incorrect Usage of Callable Objects: If you’re passing a callable object, such as a function, to timeit, ensure that you include the parentheses after the function name. Omitting the parentheses can confuse Python and trigger the error.
- Parameter Mix-Up: It’s easy to get confused about the order of arguments when calling the timeit function. Make sure you’re passing your code snippet or callable object as the first argument and any setup code as the second argument.
- Syntax Errors or Typos: Sometimes, the error might stem from a simple syntax error or typo in your code snippet. A missing colon, an extra parenthesis, or a typo in a variable name can all lead to this error. Take a closer look at your code to spot any potential mistakes.
Practical Example of Timeit Trouble
To better understand how to use the timeit module correctly, let’s walk through a practical example:
import timeit
# Define a function to be timed
def my_function():
return sum(range(100))
# Time the function
time_taken = timeit.timeit(my_function, number=1000)
print("Time taken:", time_taken)
In this example, we’re timing the execution of the my_function
function using the timeit module. The timeit.timeit()
function takes two main arguments: the code snippet or callable object to be timed (my_function
in this case) and the number of times to execute it (number=1000
).
Also Read:
Tips for Troubleshooting: Python’s Timeit Trouble
If you’re still encountering the “stmt is neither a string nor callable” error after double-checking your code, here are some additional tips to consider:
- Check for Indentation Errors: In Python, indentation matters. Make sure your code is properly indented, especially if you’re using multiple lines or nested structures.
- Review Documentation: Take a moment to review the official Python documentation for the timeit module. It provides detailed explanations and examples that can help clarify any confusion.
- Use Print Statements: Insert print statements at various points in your code to debug and track the flow of execution. This can help pinpoint the source of the error more accurately.
FAQs (Frequently Asked Questions) related to Timeit Trouble
Q: I’m getting the “stmt is neither a string nor callable” error, but I’m sure my code is correct. What could be causing it?
A: Even seasoned Python developers can fall victim to this tricky error. Double-check your code for any syntax errors, typos, or missing quotes or parentheses. It’s often the little things that trip us up!
Q: Can you give an example of how to use the timeit module correctly?
A: Certainly! Here’s a simple example:
pythonCopy codeimport timeit
# Define a function to be timed
def my_function():
return sum(range(100))
# Time the function
time_taken = timeit.timeit(my_function, number=1000)
print("Time taken:", time_taken)
Q: Is there a way to avoid using the timeit module altogether?
A: While the timeit module is handy for quick and dirty timing experiments, you can also use other methods for benchmarking and profiling your code, such as the time
module or specialized profiling tools like cProfile
Conclusion
In conclusion, the “stmt is neither a string nor callable” Python’s timeit trouble might initially seem daunting, but with patience and a systematic approach, you can overcome it. By understanding the requirements of the timeit function and carefully reviewing your code for common mistakes, you’ll be well-equipped to troubleshoot and resolve this error effectively. So, fear not, fellow Python programmer, and may your coding adventures be error-free!