cv2.error: OpenCV(4.5.2): Working with OpenCV can sometimes feel like wrestling with a stubborn octopus—an octopus that occasionally throws obscure error messages your way. One of these pesky errors is the infamous “cv2.error: OpenCV(4.5.2) XXX\shapedescr.cpp:315: error: (-215:Assertion failed) npoints ≥ 0
“. If this error has left you scratching your head, you’ve come to the right place. Let’s dive into the nitty-gritty of what this error means, why it occurs, and most importantly, how to fix it. And don’t worry, we’ll sprinkle in a bit of humor to keep things light.
Understanding the Error Message: cv2.error: OpenCV(4.5.1)
Before we jump into solving the cv2.Error, it’s important to understand what this error message is trying to tell you. The message itself is quite the mouthful:
cv2.error: OpenCV(4.5.2) XXX\shapedescr.cpp:315: error: (-215:Assertion failed) npoints ≥ 0
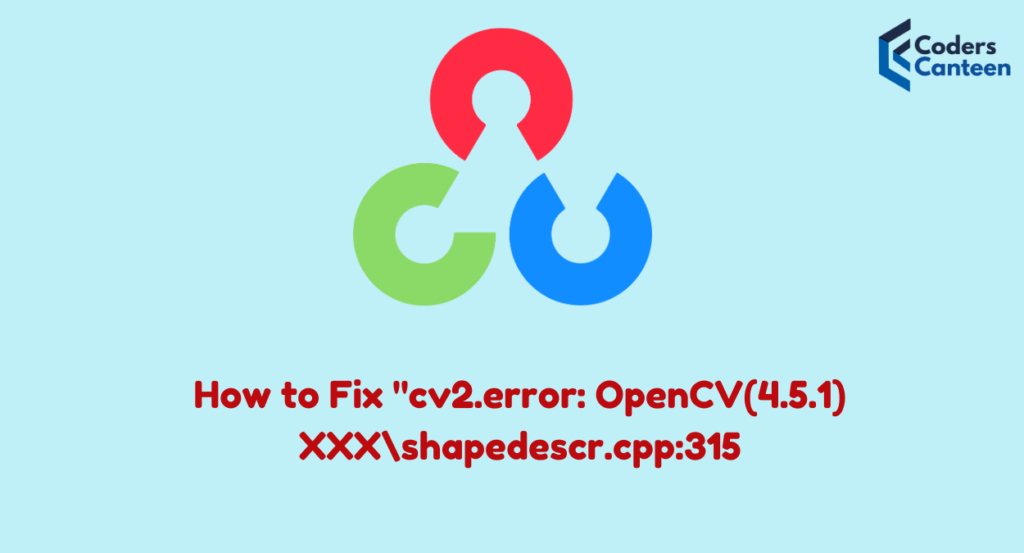
Let’s break this down about cv2.Error:
- cv2.error: This indicates that the error originates from the
cv2
module, which is OpenCV’s interface for Python. - OpenCV(4.5.1): This specifies the version of OpenCV you are using. Errors can sometimes be version-specific, so this is useful information.
- XXX\shapedescr.cpp:315: This points to the file and line number in the OpenCV source code where the error occurred. Unless you’re planning to dive into the OpenCV source code (which is a whole different adventure), this part is more useful for the OpenCV developers than for you.
- error: (-215:Assertion failed) npoints ≥ 0: This is the crux of the message. It tells us that an assertion failed because
npoints
is not meeting the condition of being greater than or equal to 0.
Translation: The error occurs because somewhere in your code, a function that requires a non-negative number of points (npoints ≥ 0
) is being given an invalid input.
Common Causes of the Error
Now that we know what the error means, let’s look at some common causes:
- Empty Contour List: This error often occurs when you pass an empty list of points to a function that expects a non-empty list.
- Invalid Image Data: If the image you are processing does not contain the expected data (e.g., if it’s all zeros or if it’s not loaded correctly), functions that rely on detecting features may fail.
- Incorrect Function Parameters: Passing incorrect parameters to OpenCV functions can also trigger this error. For instance, incorrect contour indices or hierarchy levels can cause issues.
- Improper Data Types: Ensure that the data types of the inputs match what the OpenCV functions expect.
Solving the Error: cv2.error: OpenCV(4.5.2)
Let’s go through some practical steps to fix this error. We’ll cover several scenarios and solutions.
1. Check for Empty Contours
One of the most common causes is passing an empty list of points to a function. Let’s see how we can handle this.
Example Code
import cv2
import numpy as np
# Load an image
image = cv2.imread('image.jpg')
# Convert the image to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Find contours
contours, hierarchy = cv2.findContours(gray, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# Check if any contours were found
if len(contours) == 0:
raise ValueError("No contours found in the image")
# Process the first contour
contour = contours[0]
# Ensure the contour has points
if len(contour) == 0:
raise ValueError("The contour has no points")
# Draw the contour
cv2.drawContours(image, [contour], -1, (0, 255, 0), 3)
# Display the image
cv2.imshow('Contours', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
In this example, we first check if any contours were found. If not, we raise an error with a clear message. Similarly, we check if the first contour has any points before attempting to draw it.
2. Verify Image Data: cv2.error: OpenCV(4.5.1)
Sometimes, the image might not be loaded correctly, leading to this error. Let’s ensure that the image is loaded properly.
Example Code
import cv2
# Load an image
image = cv2.imread('image.jpg')
# Check if the image was loaded correctly
if image is None:
raise ValueError("Image not loaded. Check the file path.")
# Proceed with image processing
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Further processing...
This snippet checks if the image is loaded correctly by verifying that image
is not None
. If it is, an appropriate error message is raised.
3. Validate Function Parameters: cv2.error: OpenCV(4.5.2)
Ensure that you are passing valid parameters to OpenCV functions.
Example Code
import cv2
import numpy as np
# Dummy contour for demonstration
contour = np.array([[10, 10], [20, 20], [30, 30]])
# Validate contour
if contour.ndim != 2 or contour.shape[1] != 2:
raise ValueError("Contour must be a 2D array with shape (n, 2)")
# Use contour with OpenCV function
cv2.drawContours(image, [contour], -1, (0, 255, 0), 3)
Here, we check that the contour is a 2D array with two columns, which is the expected format for most OpenCV functions dealing with contours.
4. Correct Data Types: cv2.error: OpenCV(4.5.1)
Ensure the data types of inputs match the expected types.
Example Code
import cv2
import numpy as np
# Load an image
image = cv2.imread('image.jpg')
# Convert to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Ensure data type is correct
if gray.dtype != np.uint8:
raise ValueError("Image must be of type uint8")
# Find contours
contours, hierarchy = cv2.findContours(gray, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# Proceed with contour processing...
In this example, we ensure the grayscale image has the correct data type (uint8
). This is important because OpenCV functions expect specific data types.
Also Read:
Adding Humor to Error Handling
While debugging can be frustrating, a bit of humor can lighten the mood. Here are some funny comments you can add to your code:
import cv2
import numpy as np
# Load an image
image = cv2.imread('image.jpg')
# Check if the image was loaded correctly
if image is None:
raise ValueError("Image not loaded. Did you forget to download it, genius?")
# Convert to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Find contours
contours, hierarchy = cv2.findContours(gray, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# Check if any contours were found
if len(contours) == 0:
raise ValueError("No contours found. Maybe try with a better image next time?")
# Process the first contour
contour = contours[0]
# Ensure the contour has points
if len(contour) == 0:
raise ValueError("The contour has no points. Did the image scare them away?")
# Draw the contour
cv2.drawContours(image, [contour], -1, (0, 255, 0), 3)
# Display the image
cv2.imshow('Contours', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
cv2.error: OpenCV(4.5.2) – Video Guide
FAQs related to cv2. Error on OpenCV (4.5.2)
Q: What is OpenCV?
A: OpenCV (Open Source Computer Vision Library) is an open-source computer vision and machine learning software library. It contains over 2500 optimized algorithms for various vision-related tasks.
Q: Why do I get the “npoints ≥ 0” error in OpenCV?
A: This error typically occurs when a function expecting a non-empty list of points receives an empty list. It can be due to no contours being detected, invalid image data, or incorrect parameters.
Q: How can I avoid empty contours in OpenCV?
A: Ensure the input image is processed correctly and check for empty contours before passing them to functions. Validate the image loading and preprocessing steps.
Q: How do I handle different data types in OpenCV?
A: Ensure that the inputs to OpenCV functions match the expected data types. For instance, images should be of type uint8
, and contours should be 2D arrays
Conclusion
Navigating through the maze of OpenCV errors can be akin to solving a puzzle—challenging yet rewarding. In this journey to tackle the “cv2.error: OpenCV(4.5.1) XXX\shapedescr.cpp:315: error: (-215failed) npoints ≥ 0” error, we’ve dissected its meaning, explored common causes, and provided practical solutions.
By understanding the error message, we gained insights into its origin and significance. We learned to identify common culprits such as empty contours, invalid image data, incorrect parameters, and improper data types. Armed with this knowledge, we embarked on a mission to troubleshoot and resolve the error, employing a blend of code examples, humor-infused commentary, and practical advice.
Through diligent debugging and a sprinkle of humor, we’ve not only addressed the immediate issue but also empowered ourselves to tackle future challenges with confidence. Remember, in the realm of computer vision, persistence and a sense of humor are invaluable companions.