Hey there! So, you want to dive into Android development using Java, huh? That’s awesome! Whether you’re looking to build a simple app for yourself or want to develop the next big hit on Google Play, you’re in the right place.
Here’s the deal: I remember when I first got started with Android development. It was a bit overwhelming—so many tools, frameworks, and languages to learn. But once I figured out how Java fit into the puzzle, things really started clicking. Today, I’ll walk you through the key steps, tips, and resources to help you kickstart your journey.
Ready to dive in? Let’s go!
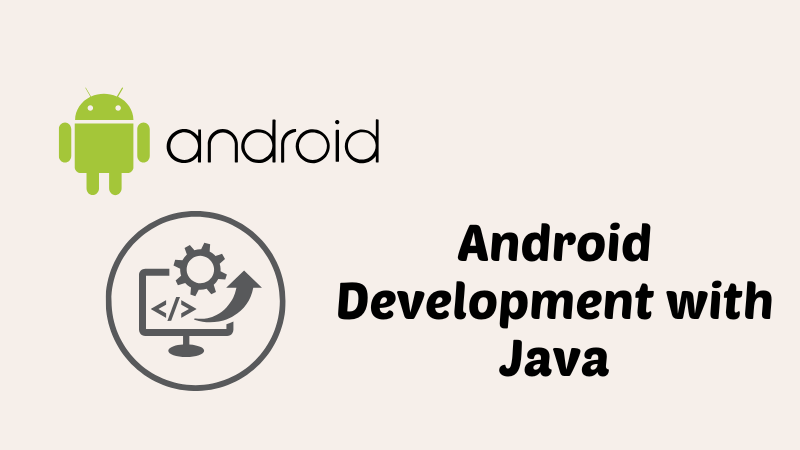
What is Android Development with Java?
Before we dive deeper, let’s make sure we’re all on the same page. Android development is the process of building apps for Android devices (smartphones, tablets, etc.). The Java programming language has been one of the primary languages for Android development for years (though Kotlin is gaining popularity). Java is powerful, easy to learn, and has a huge developer community to support you—what’s not to love?
Key Benefits of Java for Android Development
Java has some serious perks for Android development:
- Object-Oriented: It lets you structure your code in a way that’s easy to manage, especially as your app grows.
- Rich Libraries: The Android SDK (Software Development Kit) gives you access to tools that make coding faster and more efficient.
- Cross-Platform Compatibility: While Android development primarily targets Android, Java apps can be run on a variety of devices.
- Huge Community: Java’s been around for a while, so there’s a ton of tutorials, forums, and libraries out there.
How Do You Get Started with Android Development?
Here’s the thing: it might feel a little intimidating at first, but don’t worry. I promise once you get the hang of it, building Android apps with Java is incredibly fun. Let me guide you through the steps.
1. Set Up Android Studio
The first thing you need is Android Studio, the official Integrated Development Environment (IDE) for Android development. It’s packed with everything you need to start coding, including:
- Code editor
- Emulator for testing your app
- Debugging tools
- And much more
Pro Tip: Android Studio can be a bit heavy on system resources, so make sure your computer has enough power to run it smoothly.
Installation Steps:
- Download Android Studio from the official website.
- Follow the installation guide (don’t worry, it’s mostly “Next, next, next”).
- Open it up, and you’re good to go!
2. Understand the Basics of Android App Structure
Now that you’ve got Android Studio installed, let’s talk about the structure of an Android app.
An Android project consists of:
- Activities: These are screens in your app (e.g., your main menu or a settings page).
- Layouts: The XML files that define the UI of your app (buttons, text fields, etc.).
- Resources: These include images, strings, colors, and other assets used in your app.
- Java Classes: The core logic of your app. This is where you write all your functionality.
You’ll spend most of your time editing Java classes to create the logic for your app and XML files to design the UI.
How to Write Your First Java Code for Android
Let’s get coding! But before you rush ahead, take a deep breath. Learning how to write Java for Android is easier than it seems. Here’s a simple breakdown of how to start.
1. Create a New Project
Open Android Studio and create a new project. Android Studio will guide you through this process. You’ll choose:
- Project Template: For your first app, the “Empty Activity” template is perfect.
- Project Name: Something simple, like “MyFirstApp.”
- Language: Select Java.
Pro Tip: The XML layout is where you design your app, but your logic goes into the Java files. So, we’ll focus on Java for now.
2. Write Your First Java Code
Here’s a simple Java code snippet that changes the text of a button when clicked:
package com.example.myfirstapp;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button button = findViewById(R.id.myButton);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
button.setText("You clicked me!");
}
});
}
}
This simple code does the following:
- Sets up a button (with the ID
myButton
). - Listens for a click event.
- Changes the text of the button when it’s clicked.
Quick Tip: Make sure you have the correct ID for your button in the XML layout file. This ensures the button is found correctly.
Common Challenges and How to Overcome Them
Building your first app can be tricky. Don’t worry, though—I’ve faced all of these challenges too!
1. Handling Errors
You’ll definitely run into errors, especially when you’re getting started. It might seem frustrating, but here’s the thing: errors are part of the learning process. Every developer faces them.
- Tip: Google the error message! Chances are, someone else has had the same problem.
- Pro Tip: Use Android Studio’s built-in Logcat to debug your app. It will help you identify exactly where things are going wrong.
2. Learning the Android Lifecycle
The Android lifecycle might seem confusing at first, but understanding it is key to building robust apps. This lifecycle controls what happens to your app when a user opens, minimizes, or closes it.
- Tip: Get familiar with methods like
onCreate()
,onStart()
,onResume()
,onPause()
, andonStop()
.
3. Working with the User Interface
Creating a seamless and intuitive UI can be tricky, but it’s all about trial and error. You’ll want to get comfortable with Layouts, Views, and Widgets.
- Tip: Use the ConstraintLayout for flexible and responsive designs. It’s great for apps that work across different screen sizes.
Advanced Java for Android: Next Steps
Once you’ve got the basics down, it’s time to move on to more advanced concepts. Here are a few things you should dive into next:
- Working with APIs: Learn how to fetch data from external sources (e.g., weather data, social media feeds) using Retrofit or Volley.
- Data Persistence: Store data on the device using SQLite or Room (a database library).
- Building a UI with RecyclerView: This powerful component helps you display lists of data, like contacts or product catalogs.
FAQs
1. Do I need to learn Java to develop Android apps?
Yes, Java is still widely used for Android development, although Kotlin is now also popular. Java provides a solid foundation for Android development.
2. Can I use Android Studio on a Mac?
Absolutely! Android Studio is available for Windows, macOS, and Linux. Just download the appropriate version from the official website.
3. How long does it take to learn Android development?
It varies depending on your background, but with consistent practice, you can start building simple apps in a few weeks.
4. Do I need to learn XML for Android development?
Yes, XML is used to define your app’s layout and user interface. It’s an essential skill for Android developers.
Conclusion: Start Building!
Building Android apps with Java is incredibly rewarding, and once you’ve learned the ropes, you’ll be amazed at what you can create. I remember feeling a bit lost at first, but with a little patience and practice, I was able to build my first app—and it felt awesome!
So, are you ready to build your first Android app? Don’t wait! Download Android Studio, start coding, and let your creativity flow.